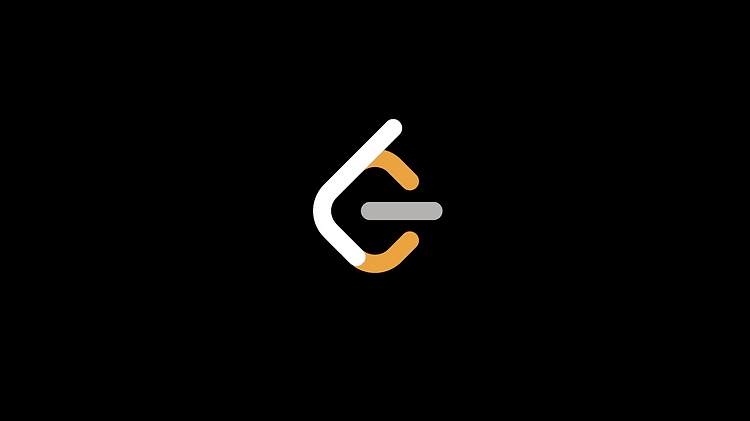
문제
Given an array of integers nums and an integer target, return indices of the two numbers such that they add up to target.
You may assume that each input would have exactly one solution, and you may not use the same element twice.
You can return the answer in any order.
예제
Example 1:
Input: nums = [2,7,11,15], target = 9
Output: [0,1]
Explanation: Because nums[0] + nums[1] == 9, we return [0, 1].
Example 2:
Input: nums = [3,2,4], target = 6
Output: [1,2]
Example 3:
Input: nums = [3,3], target = 6
Output: [0,1]
제약
Constraints:
`2 <= nums.length <= 104`
`-109 <= nums[i] <= 109`
`-109 <= target <= 109`
Only one valid answer exists.
문제 링크
LeetCode - The World's Leading Online Programming Learning Platform
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
풀이
굉장히 쉬운 문제다.
이중으로 for문을 돌며 브루트 포스로 모든 경우의 수를 확인하면 된다.
아래와 같이 값이 들어왔다고 해보자. ▼
가장 처음 값부터 시작점으로 삼아 나머지 값들과 더하며 확인한다.
3을 시작점으로 했을 때, 2와 4는 3과 합해도 target값인 6과 같지 않으므로 시작점을 다음 값으로 설정한다. ▼
2를 시작점으로 했을 때, 4는 target값인 6과 같으므로 2와 4의 인덱스를 반환하며 종료한다. ▼
C++ 코드
class Solution {
public:
vector<int> twoSum(vector<int>& nums, int target) {
vector<int> answer;
for (int i = 0; i < nums.size(); i++) {
for (int j = i + 1; j < nums.size(); j++) {
if (nums[i] + nums[j] == target) {
answer.push_back(i);
answer.push_back(j);
}
}
}
return answer;
}
};
C++이 오랜만이라 문제 푸는거 보다 메소드 이름이 length였는지 count였는지 떠올리는게 더 어려웠다.
'Algorithm > PS' 카테고리의 다른 글
백준 1065번 한수 - SWIFT, C++ (0) | 2024.02.18 |
---|---|
LeetCode 2. Add Two Numbers - C++ (0) | 2024.02.13 |
백준 1152번 단어의 개수 - SWIFT, C++ (0) | 2024.02.13 |
백준 1149번 RGB거리 - SWIFT (0) | 2024.02.13 |
백준 1074번 Z - SWIFT (0) | 2024.02.12 |